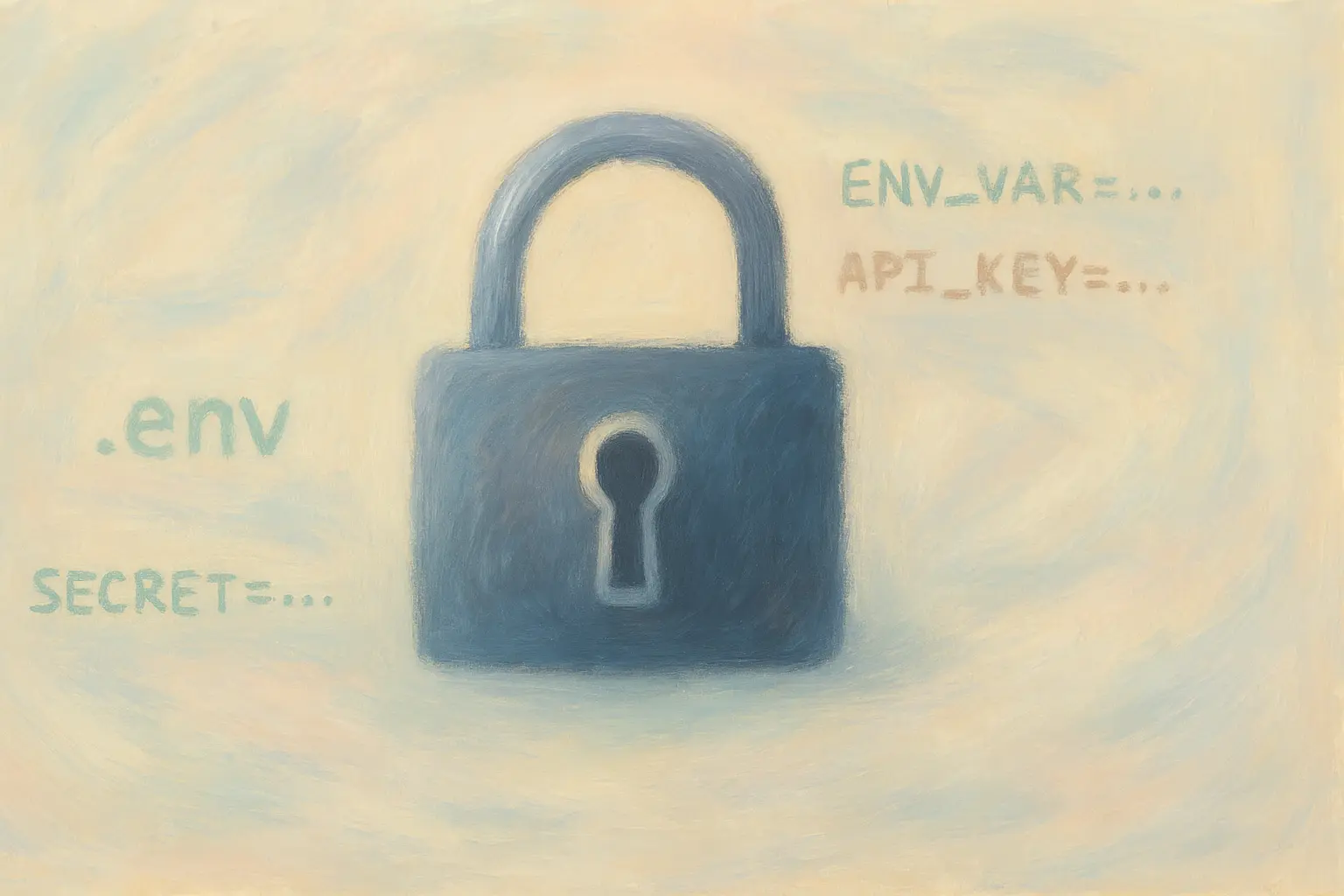
How to Keep Secrets Safe in .env Files
Table of Contents
- Why Should You Care About Secrets in .env Files?
- The Hidden Risks of Using .env Files
- Smart Practices for Securing .env Files
- 🔐 Step 1: Add .env to .gitignore
- 🔒 Step 2: Keep .env Files Out of Docker Images
- ⚔️ Step 3: Don't Hardcode Secrets in Code
- 📆 Step 4: Rotate Secrets Periodically
- 📈 Step 5: Log and Monitor Access
- 🕵️ Step 6: Follow the Principle of Least Privilege
- 🧰 Step 7: Consider Using Dedicated Secret Managers
- .env File Do's and Don'ts
- Automating Secret Injection in CI/CD
- Advanced Tips for Sensitive Projects
- Frequently Asked Questions (FAQs)
- Ready for Prime Time
Secrets like API keys, database passwords, and access tokens are the lifeblood of modern applications. But get this wrong, and you could be risking your users' data, company security, or even financial compliance. This guide is your no-fluff, developer-first blueprint to keeping environment secrets safe - especially when using .env
files.
Let's break it down in plain English, with examples, tools, and some battle-tested best practices.
Why Should You Care About Secrets in .env
Files?
.env
files help keep credentials out of your codebase. Tools like dotenv allow applications to load environment variables defined in those .env
files at runtime. It feels cleaner and separates configuration from your actual code.
But here's the catch: if mishandled, those same .env
files can end up in version control, logs, or even Docker images - open doors for attackers 🎯.
Real-world case? In 2023, New England Biolabs accidentally leaked secrets during a live stream of their development environment. Their .env
file was visible, and the rest is... security history.
The Hidden Risks of Using .env
Files
Let's talk about why .env
files (and even environment variables in general) aren't magic shields for your secrets.
1. They're Often Committed by Accident
Unless explicitly ignored in your .gitignore
file, many developers accidentally commit .env
files to Git. Yes, even experienced ones. Once pushed to a public repo, your secrets are searchable.
# .gitignore
.env
.env.* # ignores future .env.production, .env.development, etc.
2. They're Stored in Plain Text
.env
files are not encrypted. They just store values as plain text like this:
DB_PASSWORD=supersecret123
Anyone with access to the file can read its contents. Period.
3. Environment Variables Leak to Child Processes
If you spawn a new process from within your app (like running ffmpeg
, imagemagick
, or curl
), that child process inherits all the environment variables. That can expose secrets unintentionally.
4. Exposed in Logs and Crashes
Ever print process.env.MY_SECRET
for debugging and forget to remove it? If you log secrets by mistake, and logs are piped somewhere unsafe (like a shared cloud-bucket), that's a leaking bucket of trouble 🔓.
5. Visible via System Commands
On Unix-based systems, you can inspect processes using something like:
ps auxwe | grep node
Boom - plain-text environment variables. This can happen in multi-user servers, CI/CD agents, or debugging sessions.
Smart Practices for Securing .env
Files
Now that we've scared you (just a little), let's walk through how to do it right.
�� Step 1: Add .env
to .gitignore
echo ".env" >> .gitignore
This is non-negotiable. By adding .env
to your .gitignore
, you avoid accidentally committing sensitive files to version control.
Still, double-check with:
git check-ignore -v .env
Bonus: Provide a .env.example
For team use, create a template of your .env
with dummy values:
# .env.example
DB_USER=your_db_user_here
DB_PASSWORD=your_password_here
This shows what variables exist, without exposing real secrets. It's also CI/CD friendly.
🔒 Step 2: Keep .env
Files Out of Docker Images
When building Docker containers, don't add .env
files directly in your image, especially if your Dockerfile looks like this:
COPY .env /app/.env
Bad idea. Anyone who pulls the image can extract the .env
.
Safer Docker Practice
Use runtime environment variables, like so:
docker run -e DB_PASSWORD=supersecret123 my-app
Or use Docker's --env-file
:
docker run --env-file=.env my-app
This way, secrets live locally (outside the image) and are passed in during execution.
⚔️ Step 3: Don't Hardcode Secrets in Code
It's tempting to do:
const apiKey = "sk_live_abc123";
But please don't.
Instead, read it from your environment:
const apiKey = process.env.STRIPE_SECRET_KEY;
Why? Because now you can rotate or replace secrets without touching code - and more importantly, without risking your source being leaked.
📆 Step 4: Rotate Secrets Periodically
Even with the perfect secret setup, secrets don't age well. If they leak and you don't know it, attackers can access your systems indefinitely.
Best practice: Rotate secrets every 90 days
Automating this is easier if you use secret managers like:
Most of these offer automatic rotation rules that make regular updates seamless.
📈 Step 5: Log and Monitor Access
Monitoring helps you catch leaks before they become breaches.
Tools for Secrets Monitoring:
Tool | What It Does |
---|---|
Splunk | Monitors system access and logs |
ELK Stack | Centralizes log data for auditing |
Datadog | Monitors env values, app behavior, and flows |
Snyk | Scans code and dependencies for secret leaks |
GitGuardian | Tracks secret leaks in Git repos in real time |
Set up alerts for:
- Unusual access patterns
- Unexpected secrets access
- Failed reads that might signal probing
🕵️ Step 6: Follow the Principle of Least Privilege
Every part of your system should have only the permissions it needs - no more, no less. That includes environment variables.
For example:
- If your app doesn't need access to AWS S3, don't set an S3 key in its
.env
. - Frontend code should never touch secrets. Never. Ever.
Modern frameworks like Next.js use prefixing to control exposed vars:
Variable Prefix | Behavior |
---|---|
NEXT_PUBLIC_ |
Included in the browser bundle |
No prefix | Accessible only in the server |
You can see how this could go wrong:
NEXT_PUBLIC_STRIPE_SECRET_KEY=abc123 # NOPE ❌
The secret goes to the browser. Not good.
🧰 Step 7: Consider Using Dedicated Secret Managers
This is where things get enterprise-ready. Reality is, .env
files are fine for development and staging, but you should avoid using them for production.
Here's a table of popular secret managers:
Tool | Highlights |
---|---|
HashiCorp Vault | Open-source, supports dynamic secrets, vault encryption |
AWS Secrets Manager | Works with Lambda, ECS, rotation, IAM integration |
AWS Parameter Store | Simpler than Secrets Manager, good for config |
Google Secret Manager | IAM roles, logging, versions |
Azure Key Vault | Integrates with Azure AD, RBAC, seamless for Azure apps |
And they all support:
- 🔁 Rotation (automated)
- 📜 Audit logs
- 🔑 Fine-grained access controls
- 🌍 Multi-region support
.env File Do's and Don'ts
Let's recap with a handy checklist.
✅ Do This | ❌ Avoid This |
---|---|
Add .env to .gitignore |
Committing .env to GitHub |
Use .env.example for templates |
Mixing config and secrets in the same file |
Use secret managers in production | Relying solely on .env in prod |
Restrict file permission to 600 | Giving global read/write permissions |
Rotate secrets every 3 months | Re-using secrets for years |
Automating Secret Injection in CI/CD
In build pipelines like GitHub Actions, GitLab CI, or Jenkins, secrets shouldn't be stored in files but injected as needed.
GitHub Actions
jobs:
build:
runs-on: ubuntu-latest
env:
DB_PASSWORD: ${{ secrets.DB_PASSWORD }}
steps:
- uses: actions/checkout@v2
- run: echo "${DB_PASSWORD}" | some_secure_command
→ Here, secrets.DB_PASSWORD
is stored securely in GitHub and injected only during the build step.
This reduces leak surface to the absolute minimum.
Advanced Tips for Sensitive Projects
Building in fintech, healthtech, or any app handling regulated data? You'll need more than basic secrets handling.
Here's how to up the game:
- Encrypt Secrets at Rest using something like GCP KMS or AWS KMS
- Use Hardware Security Modules (HSMs) for key storage when ultra-security matters
- Audit Access Daily using tools like SIEM (Security Information and Event Management)
- Enable MFA for DevOps Tools across CircleCI, GitHub, AWS Console, etc.
- Use Immutable Infrastructure: No manual secret injection. Use image builds + vaults.
So, if there's one thing to walk away with, it's this:
Secrets aren't configuration - they're liabilities. Handle them like toxic waste: with gloves, containment, and process.
And .env
files? They're a decent container, but only if you respect their boundaries.
Ready for Prime Time
Keep .env
for development, use secret managers for the real deal, and always rotate like your job depends on it (because it might).
Looking for next steps?
- Explore HashiCorp Vault Tutorials
- Add GitGuardian to monitor your open-source repos
- Integrate automatic secret scanning in your CI build
Security isn't a one-time effort - it's a muscle you grow every day.
Happy building. Securely. 🛡️