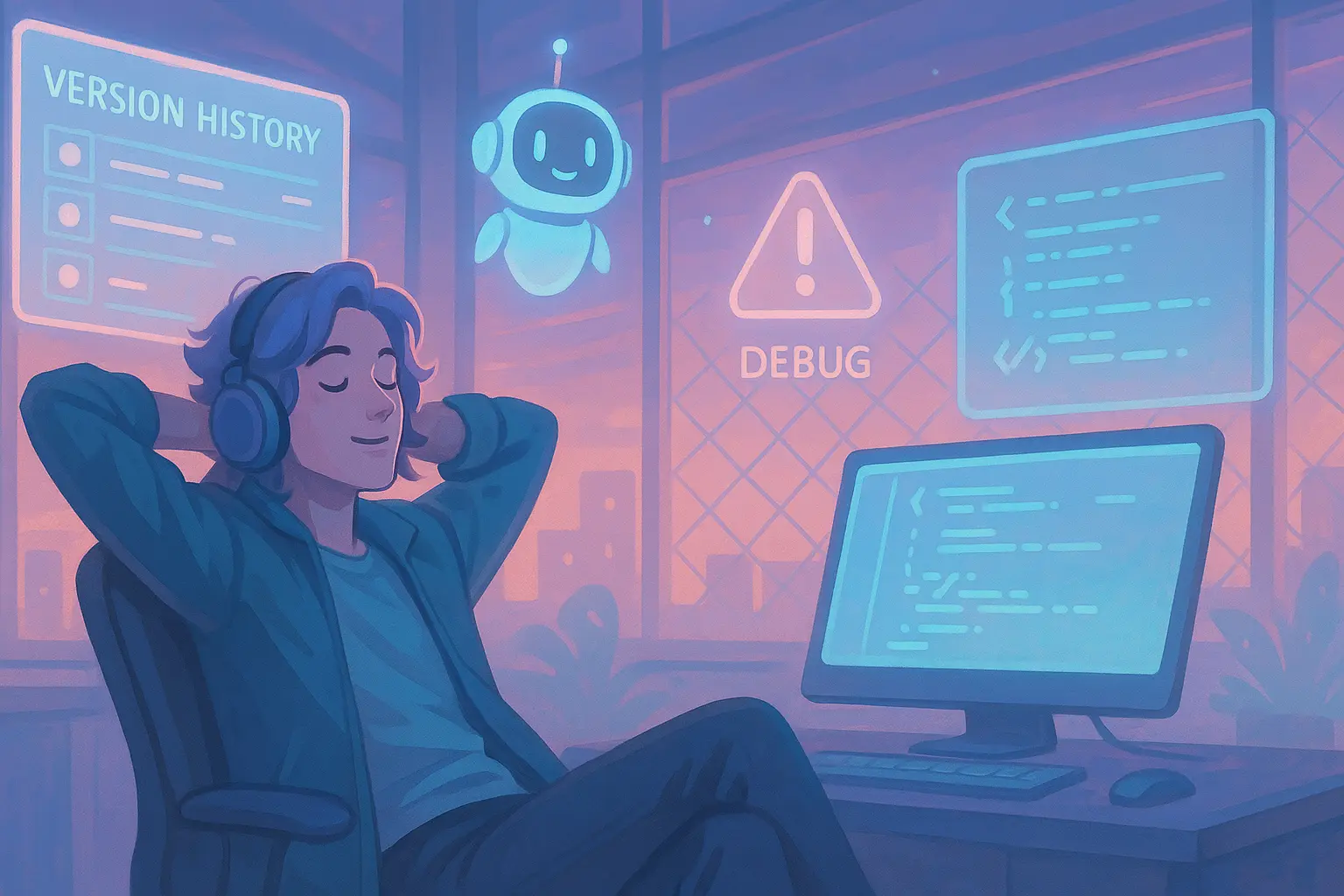
How to Save Yourself from AI Mess-Ups While Vibe Coding
Table of Contents
- Introduction
- What Exactly Is "Vibe Coding"?
- AI Adds a Whole New Layer of Complexity to Mess-Ups
- Step 1: Use Git Religiously - Even if You're Solo
- Step 2: Isolate Experiments with Branching
- Step 3: Use Git LFS and DVC for Large Model Files
- Step 4: Don't Just Print Results - Track Them
- Step 5: Automate for Consistency
- Step 6: Assume You'll Forget - Write Stuff Down
- Step 7: Create Snapshots Before Long Runs
- Step 8: Keep Your Environment Stable
- Step 9: Respect the Random Seed
- A Real Mess-Up Story: What Not to Do
- Summary Table: Vibe Coding Survival Kit
Introduction
Imagine sinking into your chair; music is playing, you're coding an AI model, and everything feels right. You're in the zone. You add some new layers, tweak a few hyperparameters, slap in a last-minute augmentation pipeline, and... run it.
Boom.
Accuracy plummets. The model starts behaving oddly. Your dataset somehow disappears. You try to undo changes, but you're not sure how far back you need to roll. Your smooth vibe is now a full-blown panic attack.
Vibe coding - that freewheeling, let's-see-what-happens style of programming - is fun, creative, and often productive. But when AI is involved, especially with large, complex systems and datasets, small oversights can snowball into catastrophic mess-ups.
In this guide, we'll walk through how to protect yourself and your code from being derailed by AI-related goof-ups. We'll blend structure with flexibility, helping you keep the creative spark alive without throwing caution to the wind.
What Exactly Is "Vibe Coding"?
"Vibe coding" is a slang term among developers for coding by feel. It typically means:
- Working late-night or in spurts of inspiration
- Skipping formal planning, diving headfirst into the code
- Experimenting wildly without worrying too much about structure
- Often working solo or informally with peers
In AI and machine learning projects, this might involve:
- Tuning models on a whim
- Testing new architectures or layers
- Running multiple experiments without tracking them properly
- Tweaking pre-processing or datasets and hoping for the best
Vibe coding is thrilling—but it's risky.
AI Adds a Whole New Layer of Complexity to Mess-Ups
Here's where it gets tricky. With traditional software, bugs are often deterministic. Input A gives output B. Breaks can usually be debugged logically.
In AI systems, behavior is emergent and stochastic. That means:
Challenge | Why It Matters in AI Projects |
---|---|
Model Behavior is Non-Intuitive | A small data preprocessing tweak can tank model performance |
Large File Sizes | Models and datasets often exceed Git's comfortable file size limits |
Reproducibility is Hard | Random seeds, environment differences, and data versioning make rerunning an experiment tricky |
Latency Between Action and Outcome | You may not know something broke until hours into training |
Shop-of-Horrors Dependencies | Packages like TensorFlow or PyTorch can introduce subtle version conflicts |
Managing these minefields requires strategy. Below, we unpack step-by-step systems that vibe coders can follow without killing that creative flow.
Step 1: Use Git Religiously - Even if You're Solo
It doesn't matter if you're coding on a hammock with no one else watching. Git is your safety rope.
Core Git Practices to Protect Your Flow
Task | Git Command |
---|---|
Start a new experiment | git checkout -b experiment/better-optimizer |
Save changes often | git add . && git commit -m "Tweak learning rate and dropout" |
Undo a bad commit without losing work | git reset --soft HEAD~1 |
Revert your code to an older but working state | git checkout <commit_id> |
Track deleted files and revive them | git log -- path/file.py + git checkout |
Want to go further? Introduce Git hooks to enforce testing or code formatting before commits. Helps keep things neat even during bursts of chaos.
Step 2: Isolate Experiments with Branching
One huge vibe killer is realizing you broke the only working version of your pipeline and can't find the bug.
Branches let you explore ideas without wrecking your core.
Branching for AI Experiments
- Main (
main
): Your stable version - Experiment branches: Wild ideas go here -
experiment/bidirectional-lstm
,experiment/augment-blur-tests
- Bugfix/hotfix branches: Found an issue in main? Create
bugfix/remove-data-leak
and patch it
Pro Tip: Always branch before vibe coding. Even if your change is just "ten lines."
Step 3: Use Git LFS and DVC for Large Model Files
If you're saving trained models using Git and you're not using Git LFS, you're going to cry one day.
Why Standard Git Fails with AI Files
File Type | Problem |
---|---|
.pt , .h5 , .ckpt , .pkl |
Binary, large, and not diff-able. Bloat your repo fast |
.csv , .json datasets |
Can grow to gigabytes and clog Git history |
Image/video datasets | Even worse - hundreds of MB per commit |
How to Save Yourself
Git LFS (Large File Storage)
- Install:
git lfs install
- Track desired file types:
git lfs track "*.pt" git lfs track "*.h5"
- Add and commit like usual.
Now Git stores only pointers locally and puts actual files in external blob storage. Lightweight history, heavy files stored elsewhere = win-win.
Data Version Control (DVC)
DVC links your datasets/model weights to Git without storing heavy files in the repo:
pip install dvc
dvc init
dvc add data/train/ # Or models/my_model.h5
git add data/.gitignore models/.gitignore
git commit -m "Track data directory with DVC"
dvc push # Pushes to defined cloud storage
DVC ensures every experiment is tied to specific code, dataset, and model.
Step 4: Don't Just Print Results - Track Them
Too many vibe testers just print(f"Accuracy: {acc}")
and move on.
That works until experiment #29, when you can't remember what version of the code, data, or hyperparameters gave 90.2% accuracy.
Use MLflow to Track Experiments
MLflow is a lightweight tool that logs:
- Which code ran
- With which parameters
- Producing which metrics
- On which model file
Set up:
pip install mlflow
Code example:
import mlflow
mlflow.set_experiment("ngram-text-classifier")
with mlflow.start_run():
mlflow.log_param("ngram", 3)
mlflow.log_metric("f1", 0.86)
mlflow.log_artifact("models/ngram_model.pkl")
Now you'll never mix up which random run gave that one beautiful confusion matrix.
Step 5: Automate for Consistency
If you plan to reuse your project in the future or work with teammates later, even vibe coding benefits from minimal automation.
Use Makefile
or Shell Scripts
Don't run 10 commands to start your workflow every time. Bundle them into a script.
Example Makefile
:
train:
python preprocess.py
python train_model.py
eval:
python evaluate.py --checkpoint=models/best.pt
Now just run make train
.
Add GitHub Actions for Basic Checks
Basic CI (continuous integration) tools can catch silly errors like:
- Broken import statements
- Incorrect argument passing
- Model training returning
nan
Example GitHub Actions workflow:
name: Run AI Tests
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Python Setup
uses: actions/setup-python@v2
with:
python-version: 3.9
- name: Install Reqs
run: pip install -r requirements.txt
- name: Run Tests
run: pytest tests/
Step 6: Assume You'll Forget - Write Stuff Down
Even if you're coding just for yourself, proposal fatigue is real. You'll forget why you changed that dropout from 0.5 to 0.2.
Where to Document:
- Code comments
- A lightweight
changelog.md
- Dedicated experiment tracker (Notion, Logseq, etc.)
- Auto-logged tools like MLflow
Even a one-liner per session helps.
Step 7: Create Snapshots Before Long Runs
Before you kick off a 10-hour training session, snapshot your code and environment.
Checklist:
- Commit all changes:
git commit -am "Before long run with data trim"
- Make sure DVC has pushed models/datasets:
dvc push
- Note the expected behavior
- Log parameters (manually or using MLflow)
This makes it far easier to debug if something goes wrong after a long AF run.
Step 8: Keep Your Environment Stable
AI projects are finicky. A small change in your torch
or transformers
version can change results.
Pro Tips:
- Use
requirements.txt
orpyproject.toml
- Write
pip freeze > requirements.txt
after finalizing an environment - Use virtual environments:
python -m venv venv
- Consider Docker if rerun environments are critical
Step 9: Respect the Random Seed
Vibe coders often forget seeds because randomness feels like magic.
But if you don't control it, you'll never reproduce your best result.
Set it consciously:
import random, numpy as np, torch
def set_seed(seed=42):
random.seed(seed)
np.random.seed(seed)
torch.manual_seed(seed)
if torch.cuda.is_available():
torch.cuda.manual_seed_all(seed)
set_seed(42)
A Real Mess-Up Story: What Not to Do
Alex, an AI student, was training a text summarization model for his thesis. Overwhelmed, he relied entirely on vibe coding.
He:
- Modified data mid-stream and didn't back up
- Wiped out weights accidentally with
rm *.pt
- Couldn't tell which of the 17 scripts actually produced "the good summary"
- Forgot seed settings so his model fluctuated wildly
- Had no Git setup or backups
The result? He lost a week's work and spent his final nights rewriting experiments under stress.
The fix? After his tragedy, he added Git, MLflow, and DVC - and never coded stressfully again.
Summary Table: Vibe Coding Survival Kit
Tool/Strategy | Purpose | Example Use |
---|---|---|
Git | Code checkpointing and versioning | git commit -m "Try layer norm" |
Git LFS | Manage large model files | git lfs track "*.pt" |
DVC | Track datasets & models | dvc add data/raw/ |
MLflow | Track experiments, metrics | mlflow.log_param("dropout", 0.2) |
Seeds | Ensure reproducibility | set_seed(42) |
Branches | Safe experiments | experiment/hyperparam-tune-2 |
CI Scripts | Catch bugs early | GitHub Actions or Makefile |
Documentation | Keep track of thinking | changelog.md or Notion |
Environment Freeze | Freeze packages | pip freeze > reqs.txt |
Snapshots | Save just before big runs | Git commit + DVC push |
At the heart of it, vibe coding is about joy. Keep the spontaneity rolling… but with a parachute.
Happy experimenting, and may your models never overfit at test time.
Frequently Asked Questions
Share this article
Related Articles
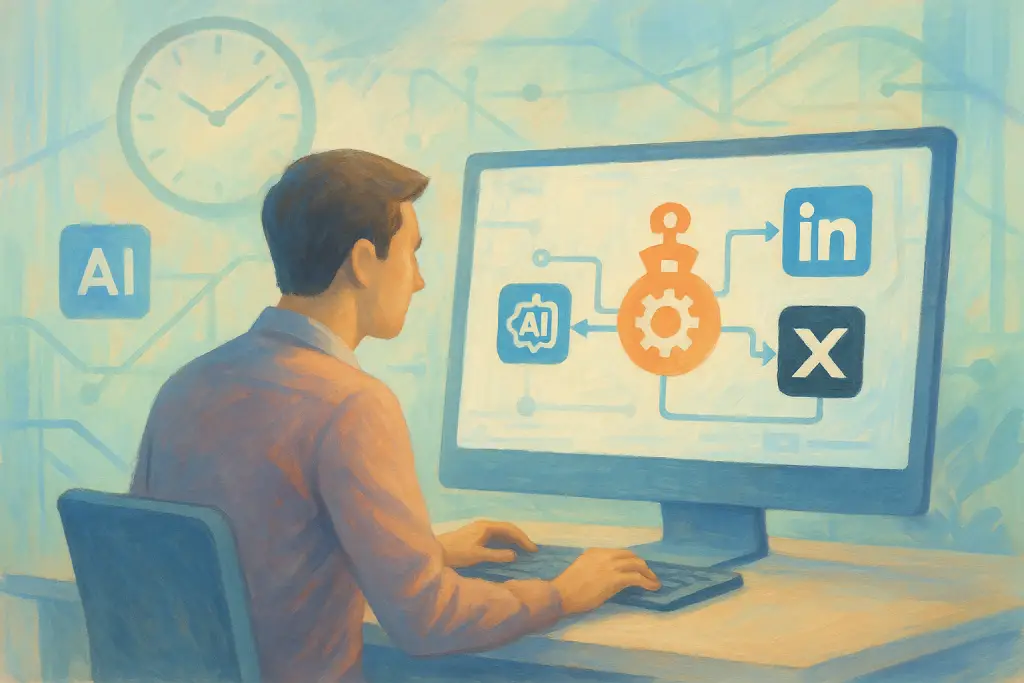
Automates Daily LinkedIn Posts from Trending AI X Posts Using n8n Workflow
Learn how to automate LinkedIn posts with AI content from X using a robust n8n workflow. The guide explains setup, benefits, component integration, and troubleshooting tips effectively.
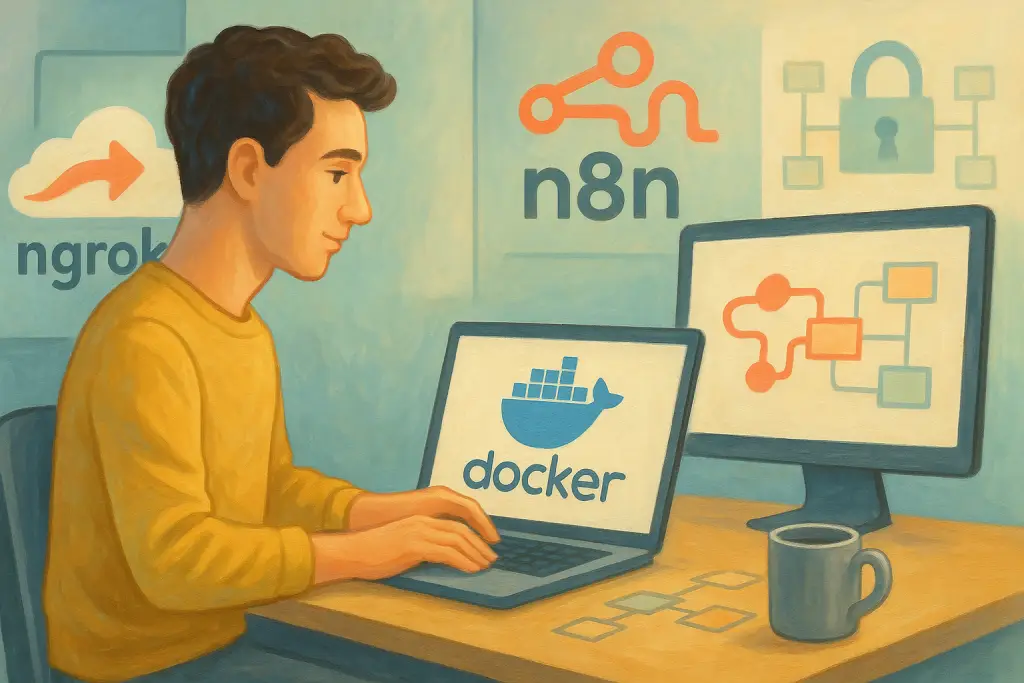
Setting Up n8n FREE Locally with ngrok for Public Access
A detailed guide to setting up the open source n8n workflow platform locally for free using Docker and ngrok for public access.
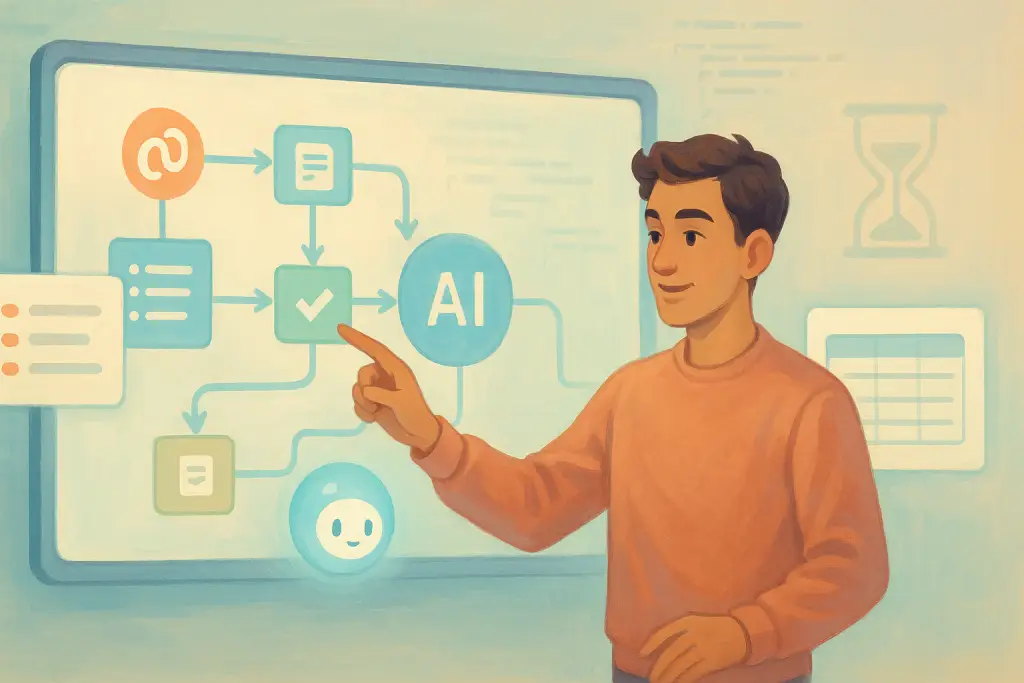
Basics of n8n Automation Workflow with AI for Beginners in 2025
A detailed and accessible guide to n8n automation workflow basics with integrated AI support for beginners in 2025, featuring practical examples, tables, and helpful lists.